使用storybook抽离公共组件
项目里一些常用的组件在复用到新项目里,总会遇到各种依赖四处流放的情况,导致我复制代码后,首先解决依赖找不到问题。寻求一种方式可以将组件的粒度缩小,不但包括项目结构方面减少依赖,还要代码灵活性上,让组件可随意配置,storybook看起来不错。
组件介绍
今天刚上手storybook,试着抽一下项目里最简单的组件:Title
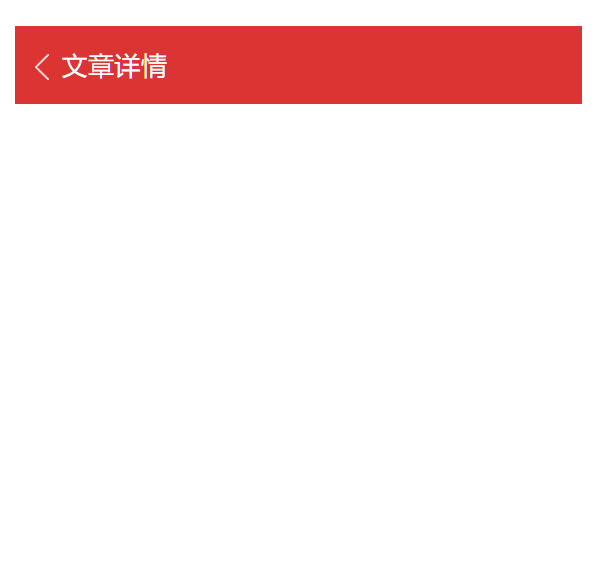
Title 用来展示手机端的标题栏,默认红色背景,白色字体,自带返回图标,点击文字或图标会返回到上一页。
这是我描述的‘组件故事’,在controls里可以对组件的props修改,并且还能收到组件的事件。
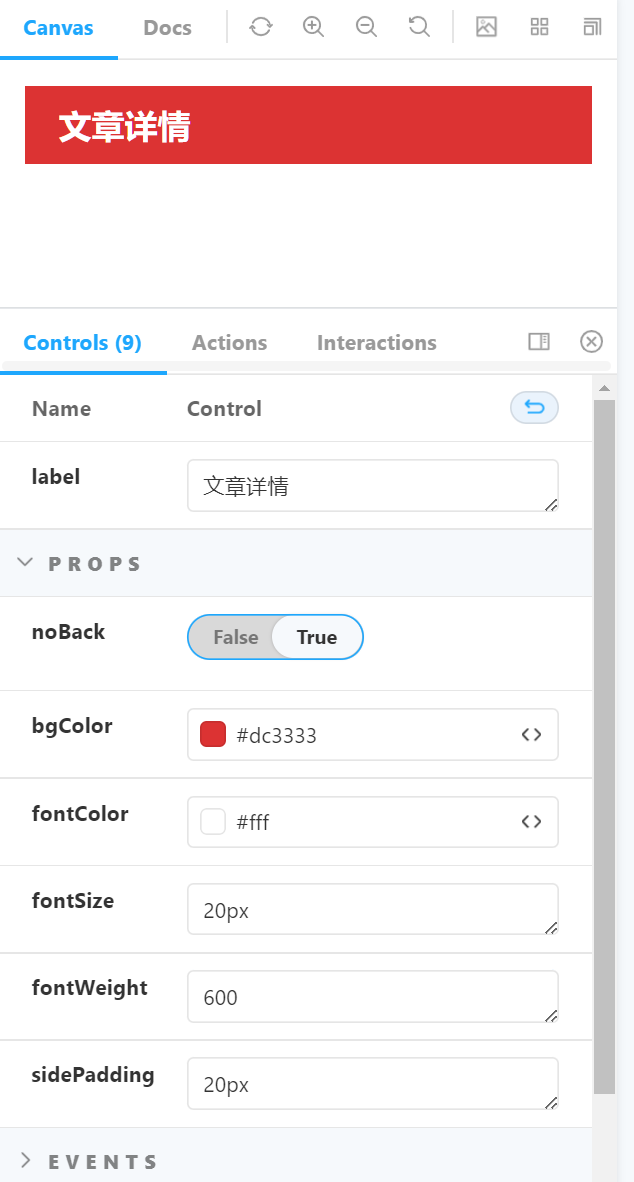
故事编写
storybook是嵌入到已存在的vue项目的,直接在项目根目录运行
1 | npx sb init |
storybook会在src里生成stories目录
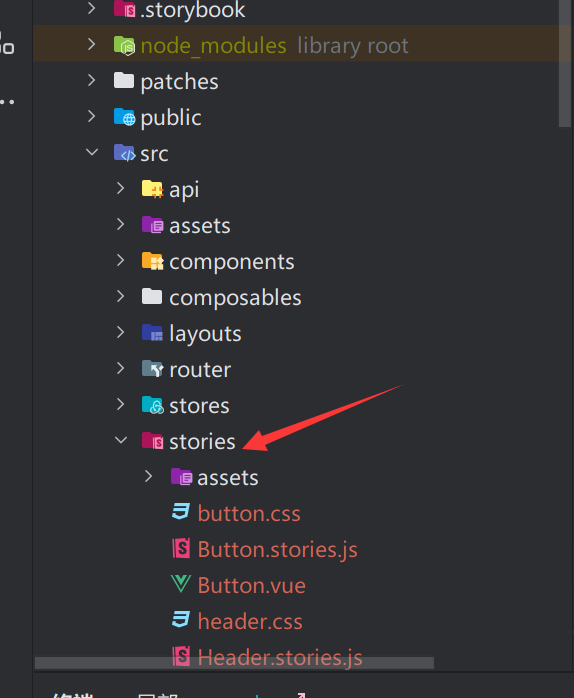
此时跑起来storybook,使用
1 | yarn storybook |
在stories里新建Title.stories.js
,将项目里的Title.vue
挪到stories里。
这是我项目里的 Title 组件
1 | <script lang="ts" setup> |
首先明显问题router
这个东西在storybook里跑不了,也就是我挪到新项目里要解决的依赖,若是将Title这个组件挪到新项目里,我还要配置一堆router,想想都头疼。
所以改造一下,换成组件内触发back事件,让调用者去决定back时干啥。
1 | <script lang="ts" setup> |
另外,组件的样式是写死的,为了让组件更灵活,我选择使用css v-bind 将props传入css里面。
1 | <script lang="ts" setup> |
组件写好了,storybook里直接引入后添加点默认配置就完成了
1 | import Title from './Title.vue' |
对,就是这么简单。
Comments
Comment plugin failed to load
Loading comment plugin