在nestjs中自定义logger
记录日志很重要,正如Apache软件基金会所说:没有错误日志的任何问题的故障排除就像闭着眼睛开车一样。
nodejs
中主流日志记录插件,如log4js
,winston
,pino
,试了log4js
,有些问题没能解决,最终听从群友建议,使用pino
。理由是:配置方便,美化日志好看!
安装
nestjs-pino 是基于pino封装的nest模块,可以拿来即用!
1 | yarn add nestjs-pino |
在main.ts
1 | import { NestFactory } from '@nestjs/core'; |
main.ts
中引入nestjs-pino
,将默认Logger
替换
继续在app.module.ts
中配置nest-pino
:
1 | import { Module } from '@nestjs/common'; |
使用useFactory
为其注入原生pino
的配置信息,为方便整理,将pinoHttp
的配置信息拎出去了
在logger.config.ts
1 | import { Request, Response } from 'express'; |
注意:保存到文件时一定要把colorize
关掉,不然有些控制字符在文件里,大概是控制颜色的字符。
插件仓库:
pinojs/pino-pretty: 🌲Basic prettifier for Pino log lines (github.com)
pinojs/pino: 🌲 super fast, all natural json logger (github.com)
nestjs-pino是pino的封装,一般它文档缺少的可以去看一下pino的,就这样。
效果
效果还ok,我的需求能看清请求信息就可以了
成功响应:
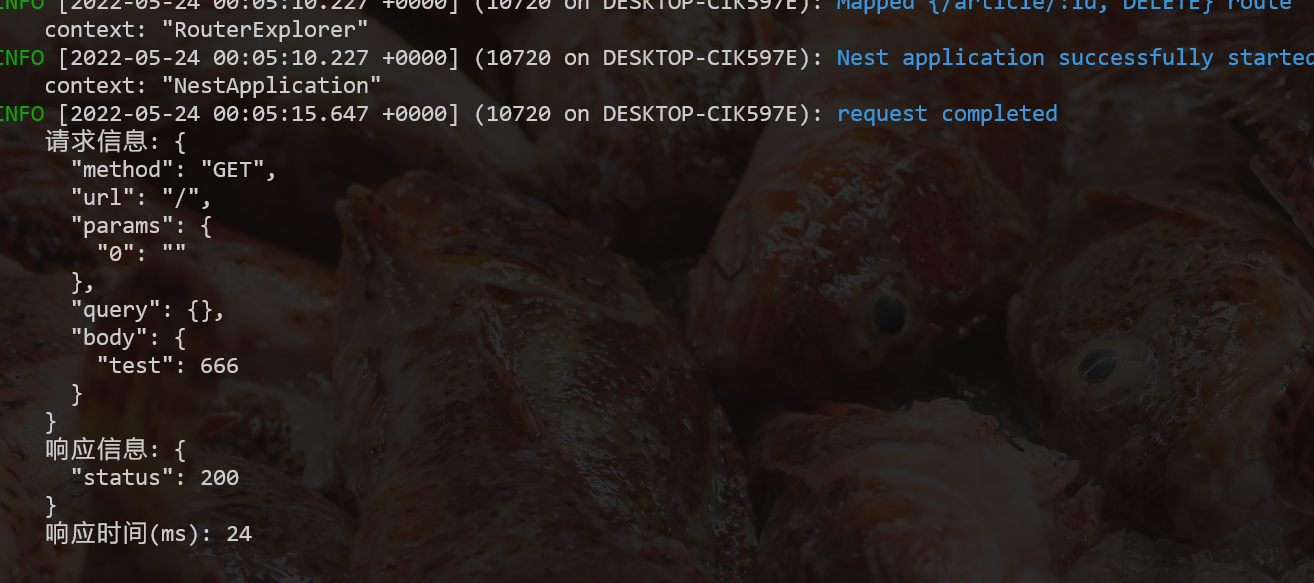
失败响应
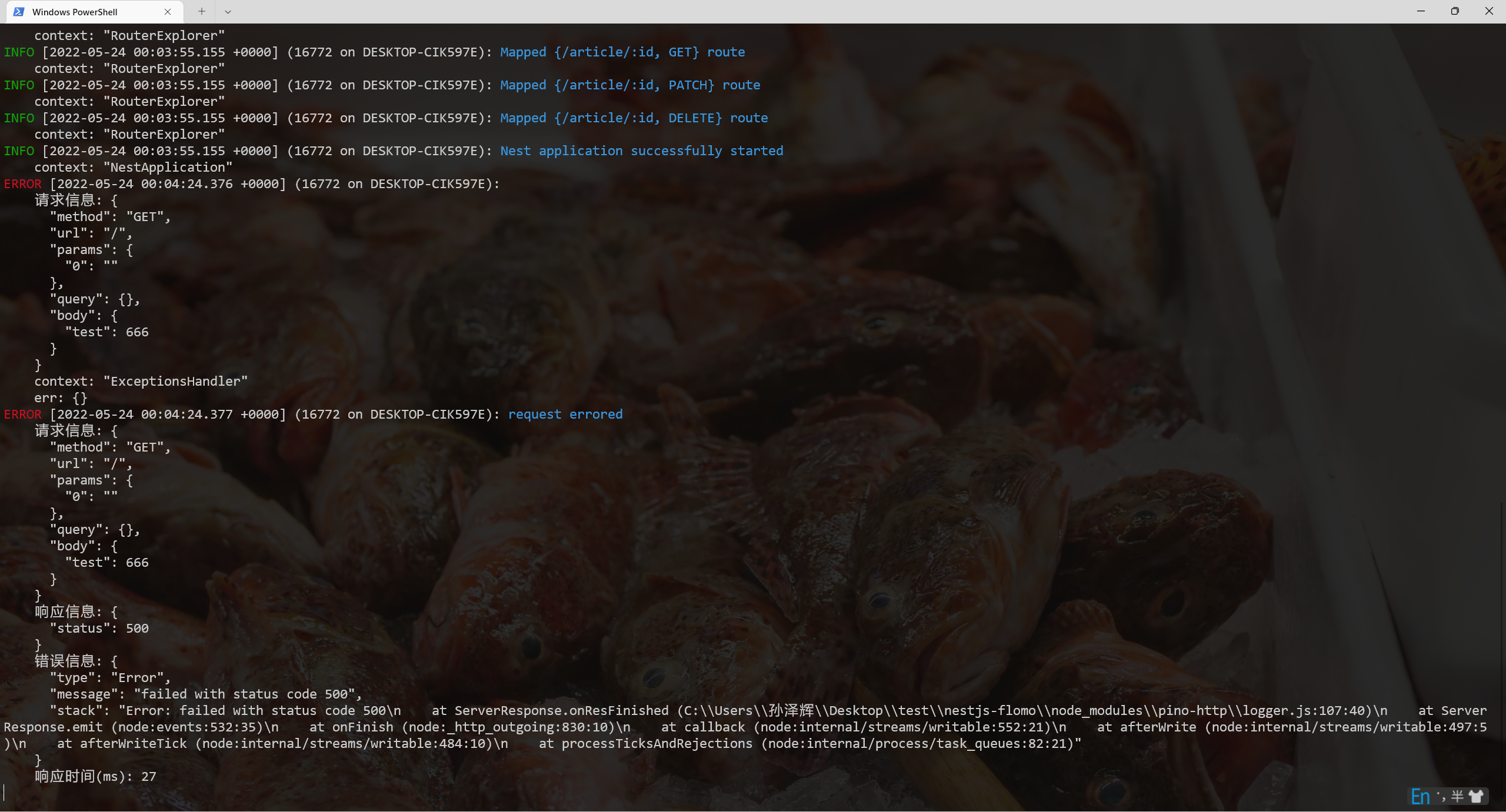
参考:
(88条消息) NestJS 7.x 折腾记: (3) 采用nestjs-pino作为Nest logger_crper的博客-CSDN博客
Comments
Comment plugin failed to load
Loading comment plugin